Blog
My notes about the "Using Node.js with Visual Studio Code" lesson
Introduction to Node.js
About Node.js
- Leverage skills with JavaScript now on the server side
- Unified development environment/language
- High performance Javascript engines - Build on Chrome's V8 JavaScript engine
- Open source, created in 2009 by Ryan Dahl
- Windows, Linux, Mac OSX
- Currently in version 8 (6 LTS)
When to use Node.js
- Node.js is great for streaming or event-based real-time applications like:
- Chat applications
- Real time applications and collaborative environments
- Game servers
- Ad servers
- Streaming servers
- Node.js is great for when you need high levels of concurrency but little dedicated CPU time
- Great for writing JavaScript code everywhere
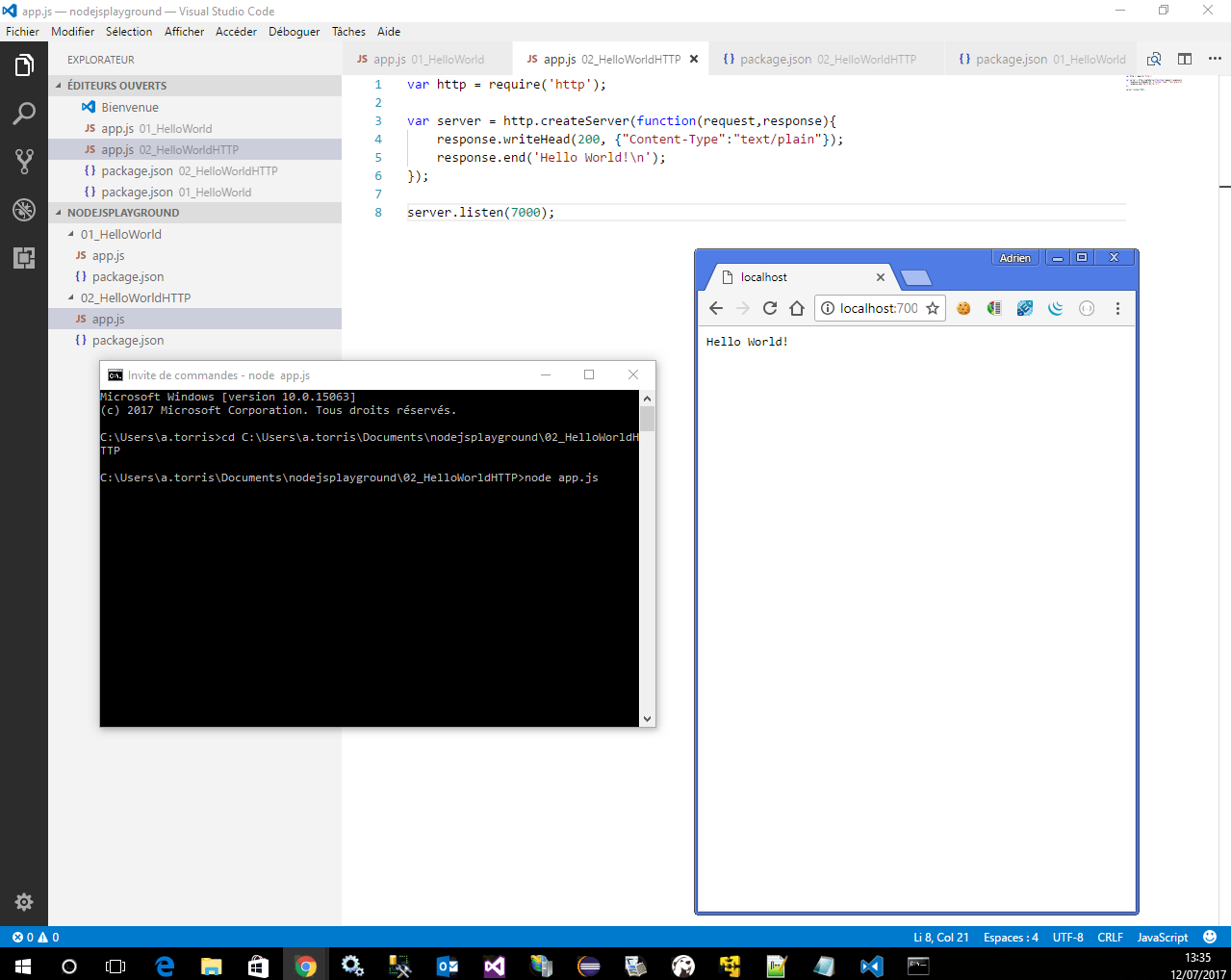
Event Driven Programming
A programming paradigm in which the flow of the program is determined by events such as user actions (mouse clicks, key presses) or messages from other programs.
Node Event Loop
- Node provides the event loop as part of the language.
- With Node, there is no call to start the loop.
- The loop starts and doesn't end until the last callback is complete.
- Event loop is run under a single thread therefore sleep() makes everything halt.
Callback Style Programming
- Event loops result in callback-style programming where you break apart a program into its underlying data flow.
- In other words, you end up splitting your program into smaller and smaller chunks until each chunk is mapped to operation with data.
- Why? So that you don't freeze the event loop on long-running operations (such as disk or network I/O).
Event Emitters
- Allows you to listen for "events" and assign functions to run when events occur.
- Each emitter can emit different types of events
- The "error" event is special
Streams
- Streams represent data streams such as I/O.
- Streams can be piped together like in Unix
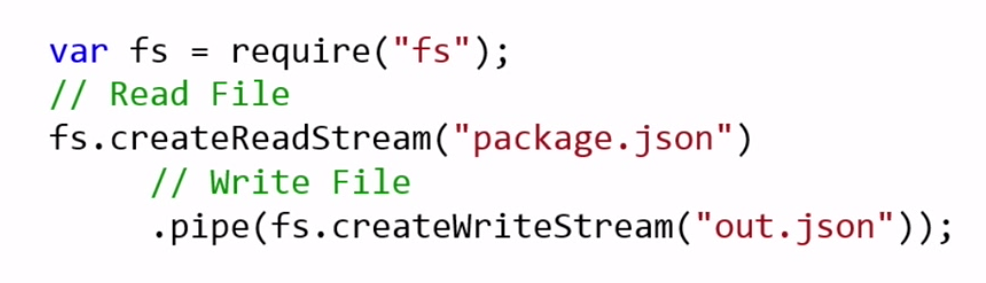
Modules and Exports
- Node.js has a simple module and dependencies loading system
- Unix philosophy -> Node philosophy ||
- Write programs that do one thing and do it well -> Write modules that do one thing and do it well. - Call the function "require" with the path of the file or directory containing the module you would like to load, and it returns a variable containing all the exported functions.
Node Package Manager (NPM)
What is NPM ?
- Official package manager for Node.
- Bundled and installed automatically with the environment.
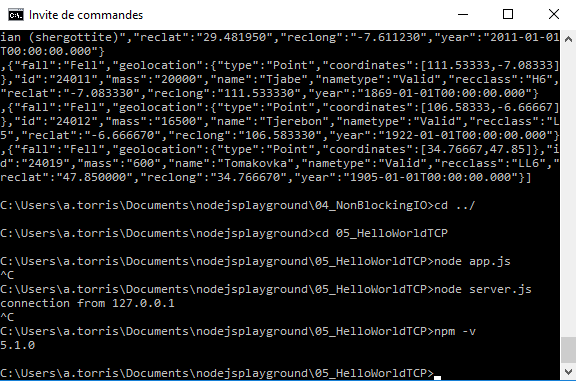
How does it work ?
- Installs the dependencies in the local node_modules folder
- In global mode (vs local mode), it makes a node module accessible to all
- Can install from a folder, tarball, web, ...
- Can specify dev or optional dependencies
- Can also install the dependencies for a project by reading the package.json
Introduction to Express
What is Express ?
Express is a minimal, open source and flexible node.js web app framework designed to make developing websites, web apps and APIs much easier.
It's a very popular framework in the Node.js community.
Why use Express ?
- Express helps you respond to requests with route support so that you may write responses to specific URLs
- Supports multiple templating engines to simplify generating HTML
Little demo
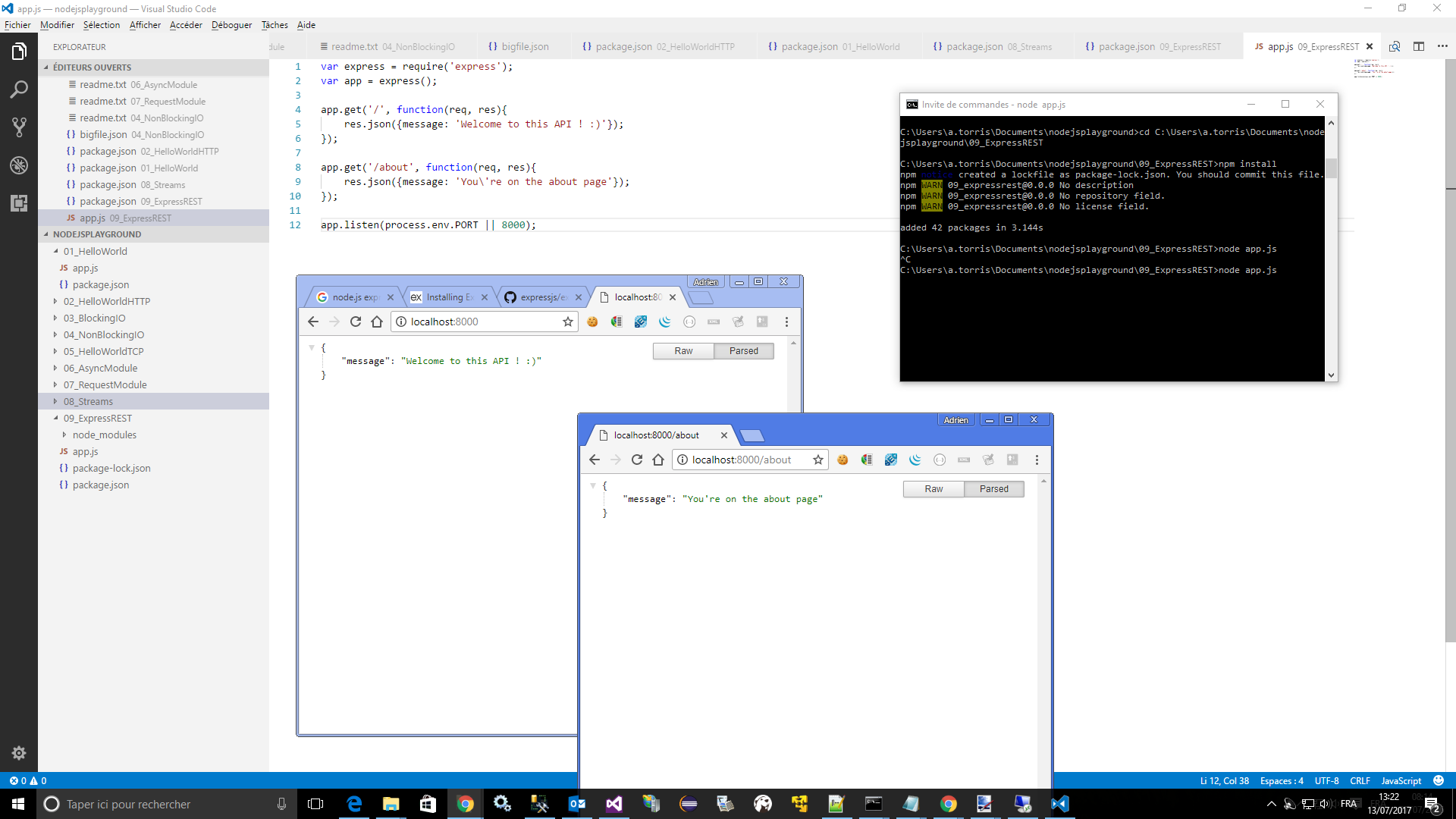
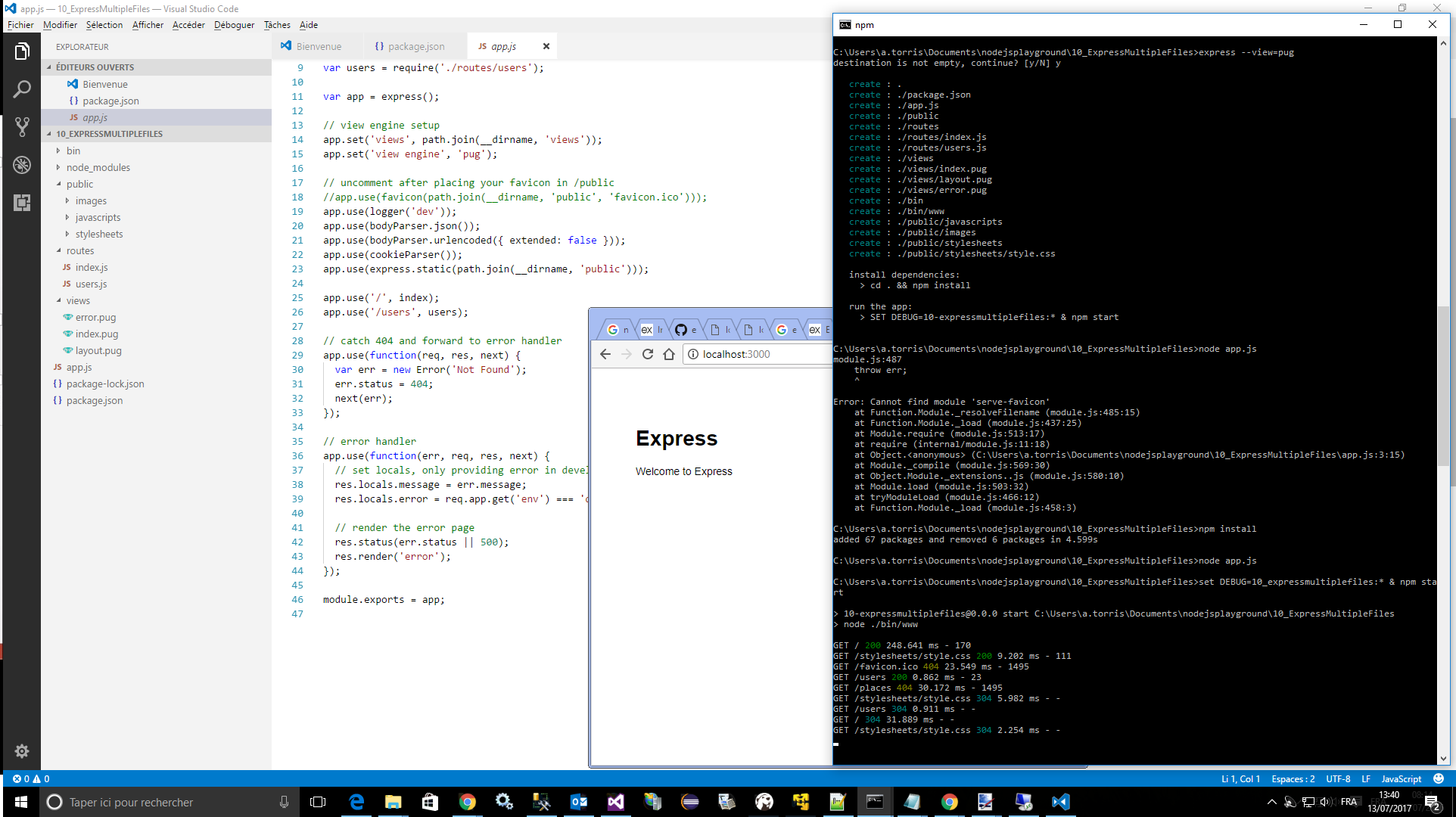
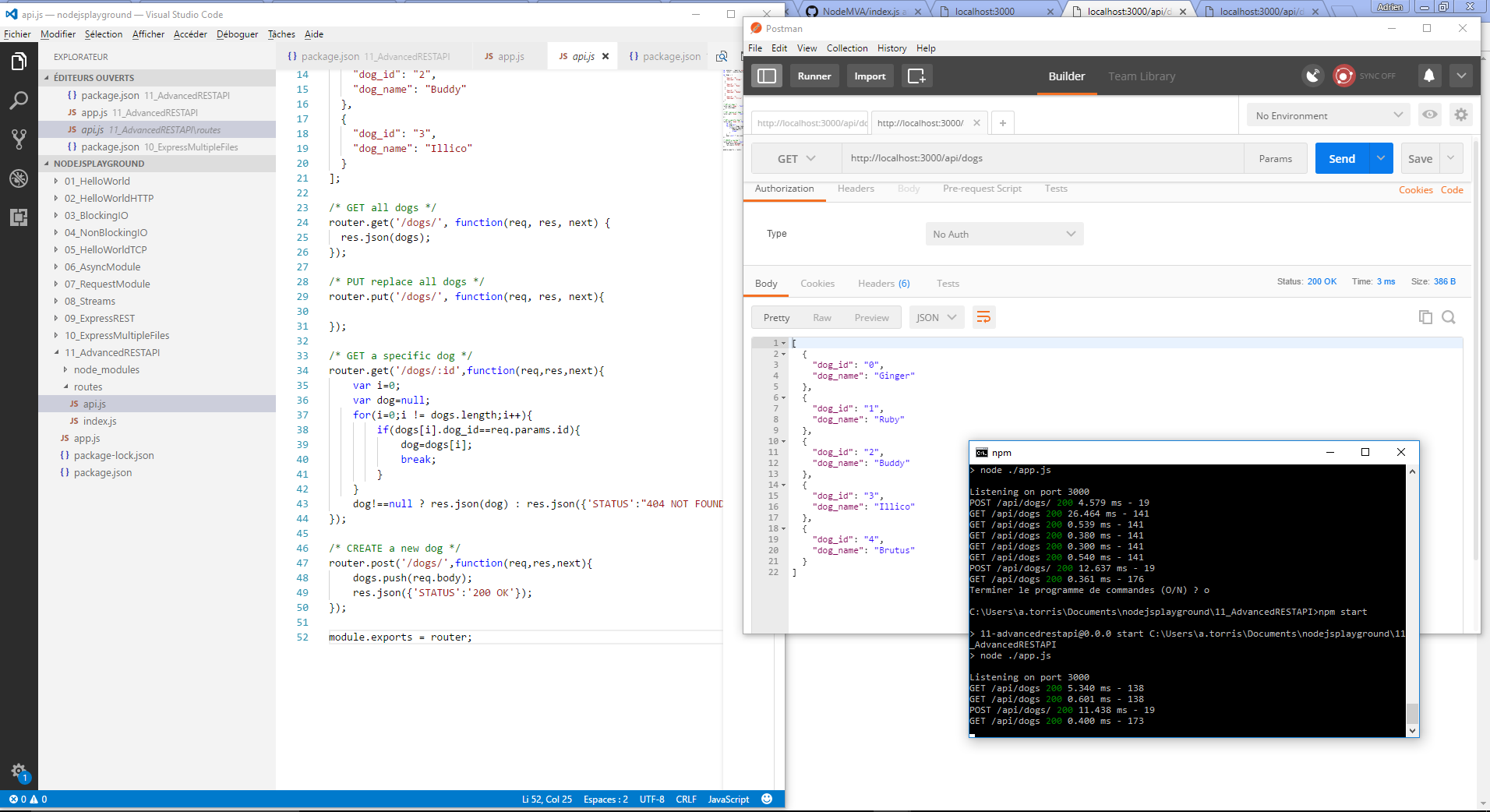
Express and Databases
About NoSQL databases
The NoSQL databases are an alternative to the relational databases. In a relational database, you have multiple tables who contains data who are different but can be joint with keys, and the model needs to be a detailed (fine-grained) database model. In the last couple of years, developers start to prefer more sclalable and flexible data models, a lot easier to manage. With a NoSQL database, no need to develop a detailed database model, you can start quickly, with this advantages and disadvantages. Of course, it's a really short and incomplete summary, you can find a lot of resources about this on the Internet.
- Not only SQL
- Different types: document based, graph databases, etc.
- MongoDB, Couchbase, Azure Cosmos DB, HBase, Cassandra, ...
- Object oriented APIs
- Good for large amounts of data, can be scaled
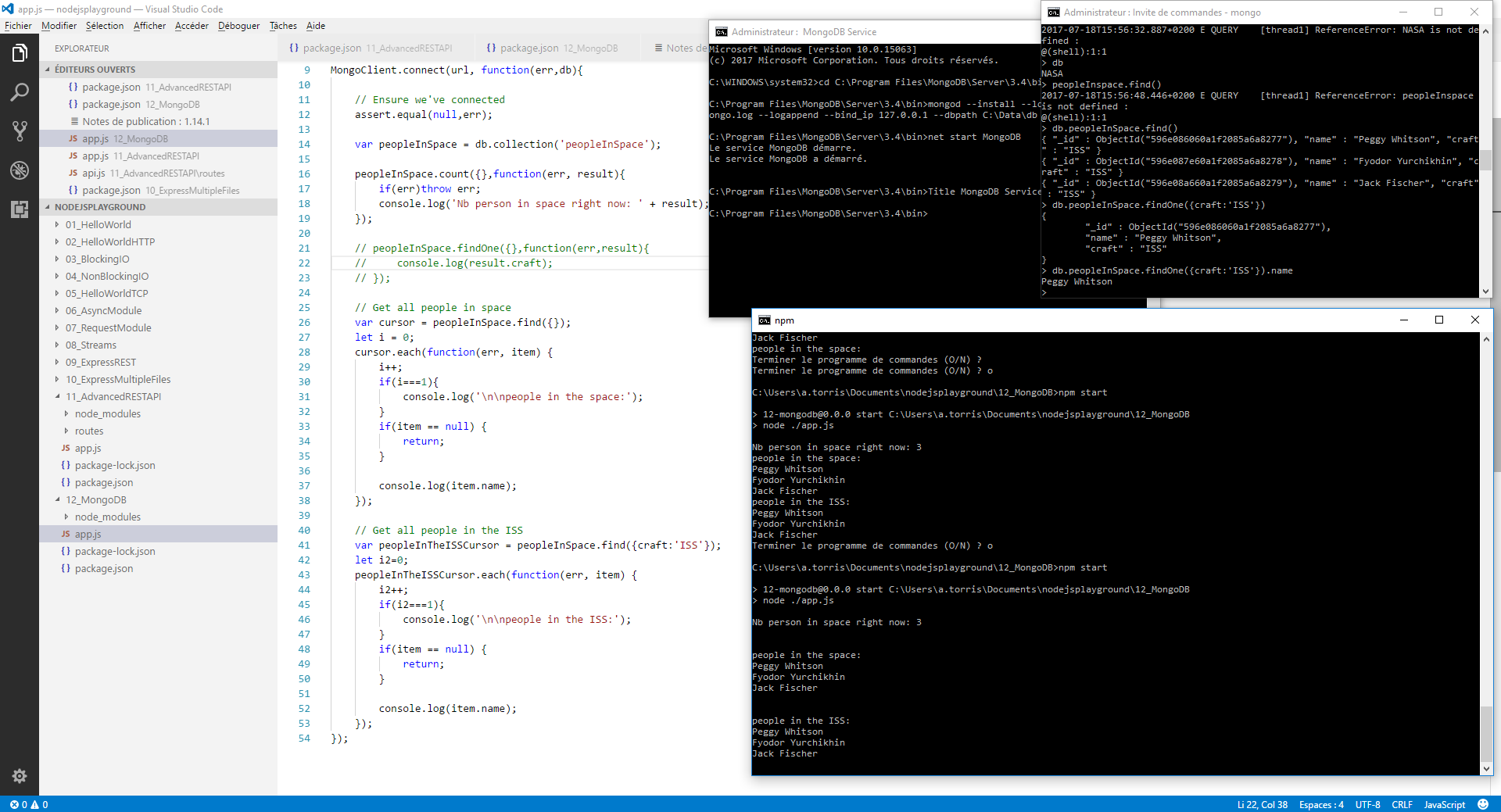
Building a Front End for your Express web apps
Notice that the lesson explains about the Jade templating engine who is now obsolete, and have been replaced by Pug.
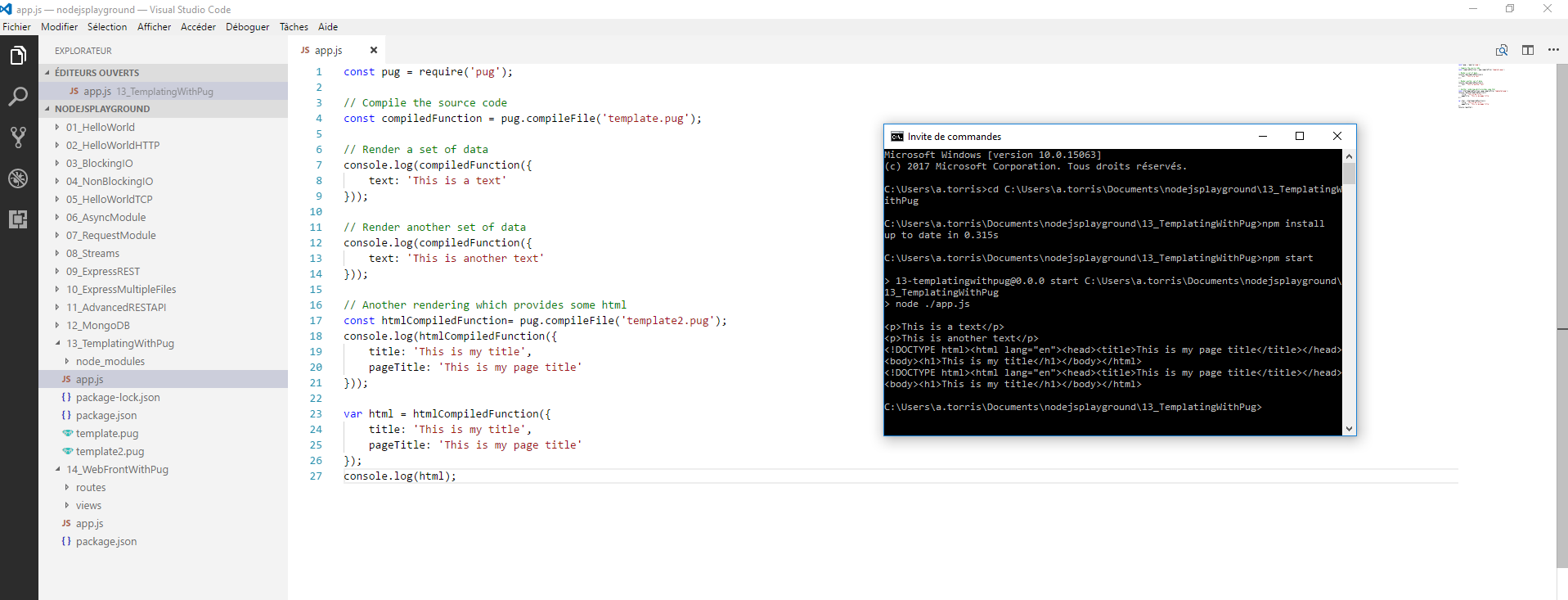
Debugging and Deploying Node.js
Azure Web Apps
- Easy to deploy a variety of different websites: node, asp.net, python, etc.
- Can install some software from the gallery like WordPress or preconfigured stacks (MEAN stacks).
- Has a few limitations such as cannot configure ports, compile native modules for Node.
- Can configure custom domains
- Can aff FTP users
- Can run multiple web sites
- Ideal for a production / staging environment
Extending Node.js with Azure Web Jobs
Azure WebJobs Overview
- Ability to run a "background task" on demand, continuously, or scheduled
- Deploy using PAAS - Part of websites
- Ideal for tasks such API aggregation, batch processing, image resizing, etc.
- Can use Node, Pythno, Bash, etc.
Indrocution to creating WebJobs for Node
- Looks for a file with "run"(run.js). If not found looks for any file with supported extension.
- Have as many files as you want, you zip them up.
- Can have multiple webjobs.
- Stored alongside your site at ./App_Data/jobs
- Using Node.js with Visual Studio Code
- Node.js
- NPM
- Node.js on GitHub
- Lesson's GitHub source code repository
- Using Node's Event Module
- Visual Studio Code
- Express
- Express on GitHub
- Express generator
- Express generator on NPM
- Express generator on GitHub
- Pug on GitHub
- Course forum
- Steven Edouard's MongoDB jump start
- Pug website