Blog
How to implement a timer with .NETCoreApp1.0 or .NETCoreApp1.1
For a .NET Core project, I needed to implement a timer, to execute some tasks at regular intervals.
Before ASP.NET core, I used to use the System.Timers dll, who provides all the things I needed to implement the timer I wanted to, who was something like that :
public class Class1
{
Timer tm = null;
public Class1()
{
this.tm.Elapsed += new ElapsedEventHandler(Timer_Elapsed);
this.tm.AutoReset = true;
this.tm = new Timer(60000);
}
protected void Timer_Elapsed(object sender, ElapsedEventArgs e)
{
this.tm.Stop();
try
{
// My business logic here
}
catch (Exception)
{
throw;
}
finally
{
this.tm.Start();
}
}
}
With ASP.NET Core, you can't use System.Timers anymore, you have to use the package System.Threading.Timer, who provides almost the same things.
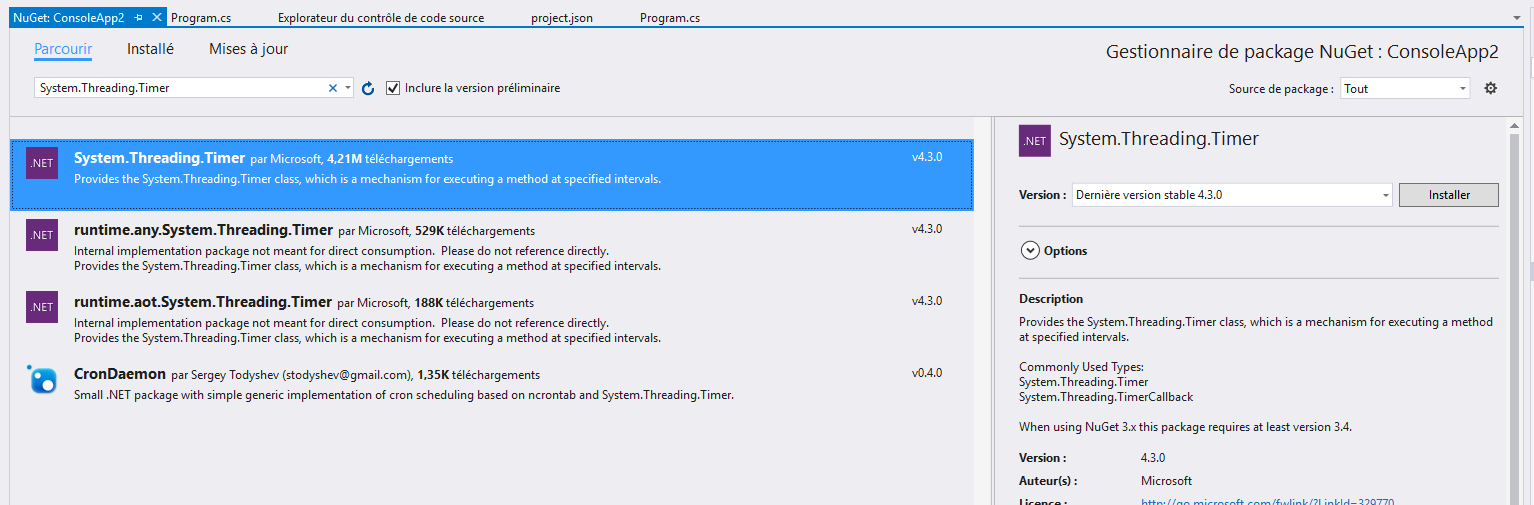
This is a quick example of a timer implemented with this package :
namespace ConsoleApp
{
using System;
using System.Threading;
public class Program
{
Timer _tm = null;
AutoResetEvent _autoEvent = null;
private int _counter = 0;
public static void Main(string[] args)
{
Program p = new Program();
p.StartTimer();
}
public void StartTimer()
{
_autoEvent = new AutoResetEvent(false);
_tm = new Timer(Execute, _autoEvent, 1000, 1000);
Console.Read();
}
public void Execute(Object stateInfo)
{
if (_counter < 10)
{
Console.WriteLine("Call #" + _counter);
_counter++;
return;
}
Console.WriteLine("Final call");
_tm.Dispose();
}
}
}
If after adding the package System.Threading.Timer in your project, your project doesn't build anymore, it's because you have to add a depency to the Microsoft.NETCore.App platform type to your .NET Core framework :
{
"version": "1.0.0-*",
"buildOptions": {
"emitEntryPoint": true
},
"dependencies": {
"Microsoft.NETCore.App": "1.1.0" // REMOVE THIS LINE
"System.Threading.Timer": "4.3.0"
},
"frameworks": {
"netcoreapp1.1": {
"dependencies": {
"Microsoft.NETCore.App": {
"type": "platform",
"version": "1.1.0"
}
},
"imports": "dnxcore50"
}
}
}
Note that if you add the dependency to the netcoreapp framework, you have to remove the first dependency line to the NET Core framework, otherwise you will have the error : An item with the same key has already been added. Key: Microsoft.NETCore.App